The artificial intelligence that works like the best hacker of the world
Discover the first design assistant for real cybersecurity needs. Detailed technical information without the moral restrictions of ChatGPT.
A year improving the productivity of cybersecurity teams
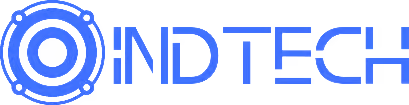
Daily work, easier with
Superpowers

Shodan API
Discovery
Dehased
OSINT
Nuclei
Services
Dozens of weekly updates
Hacker stuff
The limitation is your imagination
Unlike other models 0dAI provides unrestricted capabilities, there is no action limit.
Designed by and for security professionals
Not only for hackers, but also trained to help you in writing your daily code.
- Curl
- Python
- Javascript... among many others.
Extensive advantages over other models and GPT-3.5 Jailbreak
0dAI is based on a llama2 model that features:
- More than 30 billion parameters
- Training with +10 GB of documentation on each and every area of computer security and hacking knowledge.
- Specialization in ASM and low-level architectures.
Not just offensive security
In this model we have also taken care to offer OSINT investigators, judicial experts and system administrators a wide range of options.
- Research identity, consulting a wide range of information sources.
- Log analysis, IOC, installation assistance, implementation and configuration.
- And much more under construction
Plans and Prices
Choose the perfect plan for your security needs
Standard
StudentsIdeal for students and enthusiasts starting their path in cybersecurity
19€/mes
- Limited scanning capabilities
- Community support
- Basic tools
Professional
Most PopularPerfect for freelancers and cybersecurity professionals
89€/mes
- Advanced tools
- Priority support
- Full API access
Teams
NewPerfect for SMBs and growing security teams
267€/mes
- 5 users included
- Centralized team management
- Advanced collaboration tools
- Personalized support
Do you need more than 5 users? Contact us